Sử dụng shared_preferences trên Flutter Web
📅 — 👀 1413 — 👦Do máy mình code Flutter có cấu hình yếu nên mình debug code trên web. Nhưng khi dùng thư viện shared_preferences (nên dùng bản mới nhất) thì mỗi lần restart lại trình duyệt thì không lấy được giá trị đã lưu. Lý do là port của trình duyệt thay đổi ngẫu nhiên. Vì vậy khi debug, ta dùng hàm flutter run -d chrome --web-port 5555
trong teminal để debug và cố định port là xong.
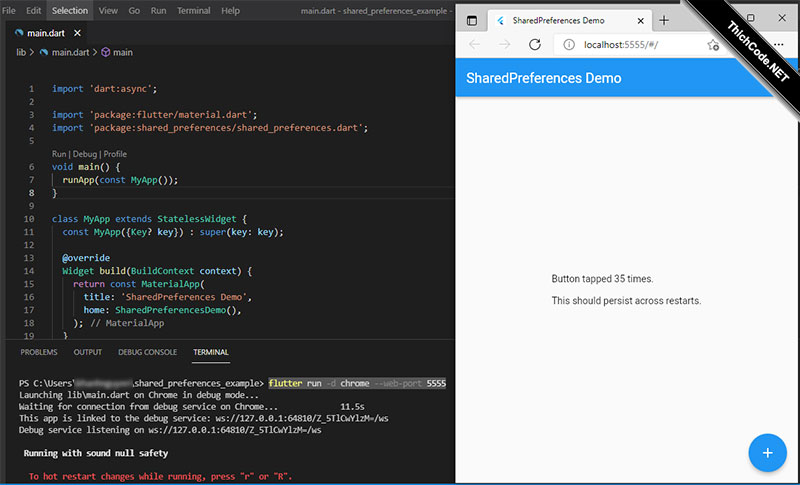
Code demo shared_preferences:
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
title: 'SharedPreferences Demo',
home: SharedPreferencesDemo(),
);
}
}
class SharedPreferencesDemo extends StatefulWidget {
const SharedPreferencesDemo({Key? key}) : super(key: key);
@override
SharedPreferencesDemoState createState() => SharedPreferencesDemoState();
}
class SharedPreferencesDemoState extends State {
final Future _prefs = SharedPreferences.getInstance();
late Future _counter;
Future _incrementCounter() async {
final SharedPreferences prefs = await _prefs;
final int counter = (prefs.getInt('counter') ?? 0) + 1;
setState(() {
_counter = prefs.setInt('counter', counter).then((bool success) {
return counter;
});
});
}
@override
void initState() {
super.initState();
_counter = _prefs.then((SharedPreferences prefs) {
return prefs.getInt('counter') ?? 0;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('SharedPreferences Demo'),
),
body: Center(
child: FutureBuilder(
future: _counter,
builder: (BuildContext context, AsyncSnapshot snapshot) {
switch (snapshot.connectionState) {
case ConnectionState.waiting:
return const CircularProgressIndicator();
default:
if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
} else {
return Text(
'Button tapped ${snapshot.data} time${snapshot.data == 1 ? '' : 's'}.\n\n'
'This should persist across restarts.',
);
}
}
})),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: const Icon(Icons.add),
),
);
}
}
📁 Flutter